Introduction to Web Scraping Challenges
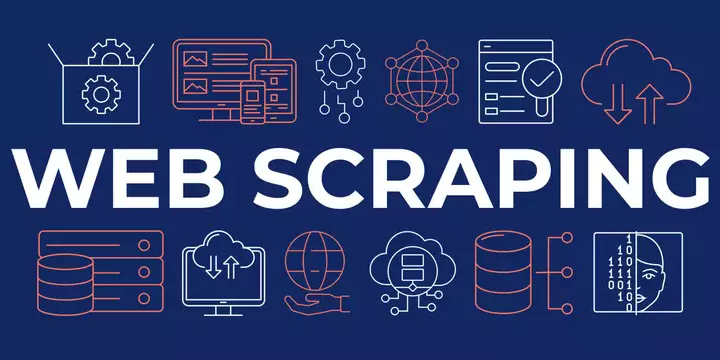
Introduction to Web Scraping Challenges
Web scraping is effective for extracting website data, but often faces rate limits and server blockages meant to safeguard data and ensure service availability. These measures can detect and block scraping, posing challenges for data collection.
Common Techniques for Overcoming Rate Limits and Blockages
1. Respecting robots.txt
Before starting to scrape, it’s crucial to check the website’s robots.txt
file, which specifies the scraping rules. Respecting these rules is essential for ethical scraping.
import requests
url = "http://example.com/robots.txt"
response = requests.get(url)
print(response.text)
2. User-Agent Rotation
Websites can block requests from non-browser user agents or from too many requests coming from the same user agent. Rotating user agents can help mimic real user behavior.
import requests
from fake_useragent import UserAgent
ua = UserAgent()
headers = {'User-Agent': ua.random}
response = requests.get('http://example.com', headers=headers)
print(response.content)
3. IP Rotation
Using proxies to rotate IP addresses can prevent servers from recognizing and blocking your scraper due to too many requests from the same IP.
import requests
proxies = {'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080', }
response = requests.get('http://example.com', proxies=proxies)
print(response.content)
4. Delay Between Requests
Introducing delays between requests can help avoid triggering rate limits, making your scraping activity appear more human-like.
import requests
import time
for _ in range(5):
response = requests.get('http://example.com')
print(response.status_code)
time.sleep(1)
# Sleep for 1 second between requests
Advanced Techniques for Sophisticated Scraping Challenges
1. Selenium for JavaScript-Loaded Sites
Some websites load their content dynamically with JavaScript. In such cases, selenium
can be used to render the page fully before scraping.
from selenium import webdriver
from time import sleep
driver = webdriver.Chrome('/path/to/chromedriver')
driver.get('http://example.com')
sleep(5)
# Wait for the page to load
html = driver.page_source
print(html)
driver.quit()
2. CAPTCHA Solving Services
CAPTCHAs are a common method to block automated scraping. Integrating CAPTCHA solving services can help bypass these challenges.
from python_anticaptcha import AnticaptchaClient, ImageToTextTask
api_key = 'YOUR_API_KEY'
captcha_fp = open('captcha.jpg', 'rb')
client = AnticaptchaClient(api_key)
task = ImageToTextTask(captcha_fp)
job = client.createTask(task)
job.join()
print(job.get_captcha_text())
3. Using Headless Browsers with Stealth Mode
Headless browsers can be detected by websites. Using stealth mode plugins or techniques can help make your scraper undetectable.
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
options = Options()
options.headless = True
options.add_argument("--window-size=1920,1080")
options.add_argument("--disable-extensions")
options.add_argument("--proxy-server='direct://'")
options.add_argument("--proxy-bypass-list=*")
options.add_argument("--start-maximized")
options.add_argument('--headless')
options.add_argument('--disable-gpu')
options.add_argument('--disable-dev-shm-usage')
options.add_argument('--no-sandbox')
options.add_argument('--ignore-certificate-errors')
driver = webdriver.Chrome(
options=options, executable_path='/path/to/chromedriver')
driver.get("http://example.com")
print(driver.page_source)
driver.quit()
4. Network Level Evasion with VPNs and TOR
Using VPNs or TOR can provide an additional layer of anonymity and IP rotation, making it harder for websites to block your scraper based on IP.
import requests
from stem import Signal
from stem.control import Controller
# Signal TOR for a new connection
with Controller.from_port(port=9051) as controller:
controller.authenticate(password='your_password')
controller.signal(Signal.NEWNYM)
proxies = {'http': 'socks5://127.0.0.1:9050',
'https': 'socks5://127.0.0.1:9050'}
response = requests.get('http://example.com', proxies=proxies)
print(response.text)
Conclusion
Overcoming rate limits and blockages while scraping websites requires a combination of respect for the website’s rules, technical know-how, and sometimes, creativity. The techniques outlined above range from simple best practices to more advanced strategies involving headless browsers, proxy rotation, and even CAPTCHA solving. Remember, ethical scraping is paramount; always ensure you’re not violating the website’s terms of service or legal regulations.
Disclaimer
This blog post is for educational purposes only. Web scraping can be legally and ethically complex. Always ensure you have permission to scrape a website and that your actions comply with all relevant laws and terms of service.